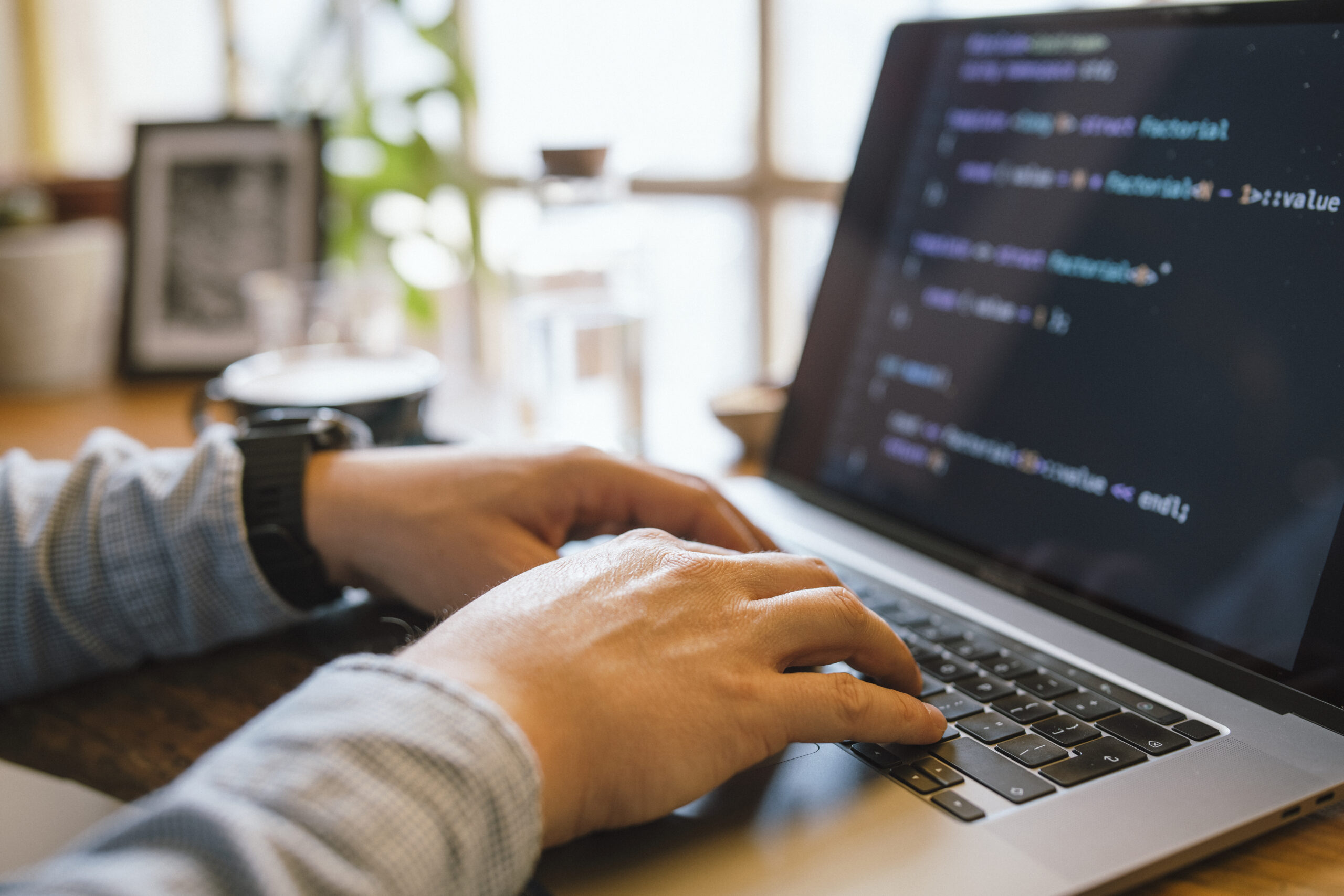
Debugging is One of the more important — nevertheless normally overlooked — abilities within a developer’s toolkit. It's actually not almost correcting damaged code; it’s about understanding how and why issues go Improper, and Understanding to Consider methodically to resolve troubles successfully. No matter if you are a beginner or a seasoned developer, sharpening your debugging abilities can save hours of frustration and dramatically improve your efficiency. Here are quite a few procedures that will help builders degree up their debugging recreation by me, Gustavo Woltmann.
Master Your Applications
On the list of fastest approaches developers can elevate their debugging skills is by mastering the applications they use everyday. When composing code is a single A part of improvement, knowing ways to communicate with it efficiently throughout execution is Similarly critical. Contemporary development environments appear equipped with impressive debugging capabilities — but many builders only scratch the surface area of what these tools can perform.
Consider, such as, an Integrated Development Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools allow you to established breakpoints, inspect the worth of variables at runtime, phase by means of code line by line, as well as modify code about the fly. When used effectively, they Allow you to notice specifically how your code behaves during execution, that's invaluable for tracking down elusive bugs.
Browser developer applications, for example Chrome DevTools, are indispensable for entrance-stop builders. They permit you to inspect the DOM, watch network requests, check out serious-time functionality metrics, and debug JavaScript in the browser. Mastering the console, sources, and community tabs can turn disheartening UI concerns into workable tasks.
For backend or process-level developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB provide deep Management over managing procedures and memory administration. Learning these equipment could possibly have a steeper learning curve but pays off when debugging functionality challenges, memory leaks, or segmentation faults.
Beyond your IDE or debugger, turn into at ease with Variation control techniques like Git to know code historical past, come across the precise moment bugs had been launched, and isolate problematic alterations.
In the long run, mastering your applications means going past default settings and shortcuts — it’s about building an intimate understanding of your growth natural environment to make sure that when challenges crop up, you’re not lost in the dark. The better you know your tools, the greater time you can spend resolving the particular challenge rather then fumbling through the procedure.
Reproduce the condition
One of the most vital — and sometimes ignored — actions in effective debugging is reproducing the problem. Right before leaping in the code or producing guesses, developers need to produce a reliable setting or situation exactly where the bug reliably seems. Devoid of reproducibility, repairing a bug gets to be a game of probability, typically leading to squandered time and fragile code alterations.
The first step in reproducing an issue is accumulating just as much context as you possibly can. Talk to issues like: What actions triggered The problem? Which atmosphere was it in — enhancement, staging, or generation? Are there any logs, screenshots, or error messages? The more element you've got, the easier it will become to isolate the exact disorders beneath which the bug takes place.
As soon as you’ve collected ample information, endeavor to recreate the issue in your neighborhood atmosphere. This may imply inputting a similar info, simulating identical consumer interactions, or mimicking procedure states. If the issue seems intermittently, consider composing automatic tests that replicate the edge conditions or state transitions included. These checks not just enable expose the problem but in addition reduce regressions Later on.
From time to time, The difficulty might be setting-specific — it might come about only on sure operating techniques, browsers, or underneath individual configurations. Employing instruments like virtual machines, containerization (e.g., Docker), or cross-browser testing platforms might be instrumental in replicating these types of bugs.
Reproducing the issue isn’t only a action — it’s a mentality. It requires persistence, observation, plus a methodical tactic. But as you can consistently recreate the bug, you are presently halfway to repairing it. By using a reproducible circumstance, You may use your debugging applications more effectively, test possible fixes securely, and talk far more Plainly with the workforce or buyers. It turns an summary criticism right into a concrete problem — and that’s exactly where developers prosper.
Browse and Have an understanding of the Mistake Messages
Mistake messages are sometimes the most useful clues a developer has when anything goes Improper. As opposed to seeing them as frustrating interruptions, builders really should study to deal with error messages as immediate communications with the technique. They usually let you know exactly what transpired, wherever it occurred, and at times even why it happened — if you know the way to interpret them.
Start off by reading through the message thoroughly and in full. Lots of builders, especially when less than time strain, glance at the 1st line and quickly start earning assumptions. But deeper in the mistake stack or logs might lie the legitimate root lead to. Don’t just duplicate and paste error messages into search engines like yahoo — read and recognize them initial.
Crack the error down into sections. Is it a syntax mistake, a runtime exception, or a logic mistake? Does it place to a specific file and line range? What module or perform activated it? These concerns can tutorial your investigation and point you towards the liable code.
It’s also beneficial to be familiar with the terminology in the programming language or framework you’re utilizing. Mistake messages in languages like Python, JavaScript, or Java often stick to predictable styles, and Studying to recognize these can greatly quicken your debugging approach.
Some faults are vague or generic, and in All those instances, it’s critical to look at the context in which the error transpired. Test related log entries, enter values, and up to date variations within the codebase.
Don’t forget about compiler or linter warnings possibly. These normally precede bigger troubles and supply hints about opportunity bugs.
Ultimately, error messages usually are not your enemies—they’re your guides. Mastering to interpret them the right way turns chaos into clarity, helping you pinpoint concerns more rapidly, lower debugging time, and turn into a extra economical and confident developer.
Use Logging Wisely
Logging is Probably the most effective equipment in the developer’s debugging toolkit. When utilised proficiently, it offers authentic-time insights into how an software behaves, serving to you fully grasp what’s occurring beneath the hood without having to pause execution or action from the code line by line.
A fantastic logging tactic commences with figuring out what to log and at what stage. Widespread logging amounts contain DEBUG, Information, WARN, Mistake, and Lethal. Use DEBUG for specific diagnostic data for the duration of growth, Information for common events (like successful get started-ups), Alert for prospective problems that don’t break the applying, ERROR for real problems, and Lethal if the method can’t continue.
Stay clear of flooding your logs with too much or irrelevant facts. Excessive logging can obscure crucial messages and slow down your procedure. Target important events, condition adjustments, enter/output values, and significant choice details within your code.
Structure your log messages Plainly and regularly. Involve context, for example timestamps, ask for IDs, and function names, so it’s much easier to trace troubles in distributed programs or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
Throughout debugging, logs Enable you to track how variables evolve, what ailments are met, and what branches of logic are executed—all devoid of halting the program. They’re In particular beneficial in generation environments exactly where stepping by way of code isn’t possible.
Moreover, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about balance and clarity. By using a perfectly-believed-out logging tactic, you can decrease the time it will require to identify problems, get further visibility into your applications, and Enhance the Total maintainability and trustworthiness of your code.
Feel Similar to a Detective
Debugging is not merely a technical activity—it is a sort of investigation. To effectively determine and correct bugs, builders will have to approach the process just like a detective fixing a thriller. This way of thinking allows break down complicated concerns into workable sections and abide by clues logically to uncover the root cause.
Begin by gathering evidence. Look at the signs of the challenge: mistake messages, incorrect output, or functionality difficulties. The same as a detective surveys against the check here law scene, accumulate just as much suitable facts as you are able to without having jumping to conclusions. Use logs, check instances, and user reports to piece alongside one another a transparent photo of what’s occurring.
Following, kind hypotheses. Question oneself: What could possibly be leading to this behavior? Have any changes recently been built to your codebase? Has this situation transpired prior to below related conditions? The aim is always to narrow down possibilities and detect probable culprits.
Then, examination your theories systematically. Attempt to recreate the problem inside of a controlled atmosphere. In the event you suspect a selected purpose or element, isolate it and validate if The problem persists. Like a detective conducting interviews, talk to your code inquiries and Allow the effects direct you closer to the reality.
Pay out shut consideration to little aspects. Bugs typically hide from the least envisioned areas—similar to a missing semicolon, an off-by-a person error, or simply a race problem. Be complete and affected individual, resisting the urge to patch The problem without entirely comprehending it. Momentary fixes might cover the actual difficulty, just for it to resurface later.
And finally, keep notes on Whatever you tried and uncovered. Equally as detectives log their investigations, documenting your debugging procedure can help save time for future troubles and assistance Other individuals fully grasp your reasoning.
By thinking just like a detective, builders can sharpen their analytical competencies, method troubles methodically, and come to be more effective at uncovering hidden troubles in advanced systems.
Compose Assessments
Producing checks is among the most effective methods to increase your debugging techniques and All round growth performance. Tests not only assist catch bugs early but additionally serve as a safety Internet that provides you self confidence when building variations towards your codebase. A well-tested software is much easier to debug mainly because it allows you to pinpoint exactly exactly where and when an issue occurs.
Start with device checks, which deal with unique capabilities or modules. These compact, isolated checks can promptly expose no matter if a certain piece of logic is Functioning as expected. When a test fails, you straight away know where by to glance, appreciably minimizing time spent debugging. Device assessments are Specially beneficial for catching regression bugs—problems that reappear following previously remaining mounted.
Up coming, integrate integration assessments and conclude-to-finish tests into your workflow. These enable be certain that numerous parts of your software perform together efficiently. They’re specifically useful for catching bugs that come about in intricate systems with various elements or services interacting. If a thing breaks, your exams can show you which Section of the pipeline unsuccessful and beneath what circumstances.
Producing tests also forces you to definitely Believe critically regarding your code. To test a element effectively, you would like to grasp its inputs, envisioned outputs, and edge circumstances. This volume of comprehension naturally qualified prospects to raised code construction and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug may be a robust first step. After the take a look at fails regularly, it is possible to deal with fixing the bug and look at your exam pass when The problem is solved. This approach ensures that precisely the same bug doesn’t return Down the road.
In short, composing assessments turns debugging from the frustrating guessing recreation right into a structured and predictable course of action—helping you catch extra bugs, faster and even more reliably.
Just take Breaks
When debugging a tough difficulty, it’s easy to become immersed in the trouble—watching your display screen for hrs, hoping Alternative after Answer. But Just about the most underrated debugging equipment is actually stepping away. Getting breaks will help you reset your head, lower annoyance, and infrequently see The difficulty from the new point of view.
When you are way too near to the code for way too prolonged, cognitive exhaustion sets in. You would possibly start out overlooking evident glitches or misreading code you wrote just hrs previously. On this state, your brain becomes fewer economical at challenge-fixing. A short walk, a espresso split, as well as switching to a special job for 10–quarter-hour can refresh your emphasis. Several developers report finding the foundation of a difficulty when they've taken time and energy to disconnect, allowing their subconscious function in the history.
Breaks also support avoid burnout, Particularly all through extended debugging periods. Sitting before a screen, mentally trapped, is not merely unproductive but also draining. Stepping absent lets you return with renewed Power in addition to a clearer frame of mind. You could instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
If you’re caught, a good guideline would be to established a timer—debug actively for 45–sixty minutes, then take a five–ten minute crack. Use that time to maneuver around, stretch, or do something unrelated to code. It might feel counterintuitive, Specially under restricted deadlines, but it really truly causes quicker and more practical debugging in the long run.
In a nutshell, having breaks just isn't an indication of weak spot—it’s a smart approach. It presents your brain Room to breathe, increases your perspective, and aids you steer clear of the tunnel eyesight that often blocks your progress. Debugging is often a psychological puzzle, and rest is a component of fixing it.
Master From Each and every Bug
Just about every bug you come upon is more than just A brief setback—It can be a possibility to develop being a developer. Irrespective of whether it’s a syntax mistake, a logic flaw, or perhaps a deep architectural concern, each can train you a little something beneficial should you make time to replicate and review what went wrong.
Start off by inquiring on your own a few important concerns after the bug is settled: What induced it? Why did it go unnoticed? Could it are already caught previously with superior techniques like device screening, code testimonials, or logging? The solutions typically expose blind spots within your workflow or knowing and allow you to Create more powerful coding behavior shifting forward.
Documenting bugs can also be an excellent habit. Continue to keep a developer journal or manage a log in which you Observe down bugs you’ve encountered, how you solved them, and Everything you discovered. Over time, you’ll begin to see designs—recurring troubles or frequent errors—that you can proactively stay clear of.
In staff environments, sharing Whatever you've realized from a bug with all your friends could be especially impressive. No matter if it’s by way of a Slack message, a brief compose-up, or A fast know-how-sharing session, aiding Other people steer clear of the very same problem boosts workforce effectiveness and cultivates a stronger Mastering tradition.
Extra importantly, viewing bugs as lessons shifts your mindset from annoyance to curiosity. As opposed to dreading bugs, you’ll start appreciating them as vital parts of your progress journey. In any case, a lot of the ideal builders will not be the ones who publish perfect code, but individuals who continuously understand from their mistakes.
Ultimately, Each individual bug you resolve provides a brand new layer to the talent set. So following time you squash a bug, have a moment to mirror—you’ll occur away a smarter, a lot more able developer because of it.
Conclusion
Increasing your debugging skills normally takes time, observe, and patience — nevertheless the payoff is big. It will make you a more productive, self-confident, and able developer. The next time you are knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become far better at Whatever you do.